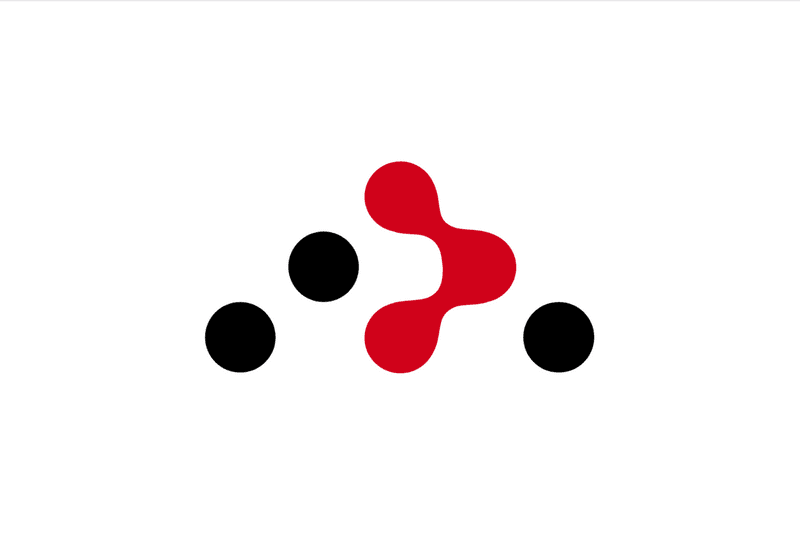
TypeScript (TS) is a statically typed superset of JavaScript that enhances the language by adding type safety, interfaces, and modern features to help developers write more reliable and maintainable code. Developed by Microsoft, TypeScript has quickly become one of the most popular tools for web development.
Why Use TypeScript?
TypeScript addresses many of the limitations of JavaScript, particularly in large-scale projects. Here are some key benefits:
-
Static Typing: TypeScript introduces static typing, allowing developers to define variable types explicitly. This helps catch type-related errors during development, reducing runtime errors.
-
Enhanced IDE Support: TypeScript's type system enables better code completion, refactoring, and error-checking in modern IDEs, making the development process smoother and more efficient.
-
Modern JavaScript Features: TypeScript includes features from the latest versions of JavaScript (ES6+), like classes, modules, and arrow functions, while also providing additional features like interfaces, enums, and generics.
-
Improved Code Maintenance: By making types explicit, TypeScript improves code readability and maintainability, making it easier for developers to understand the codebase.
How TypeScript Works
TypeScript code is written in .ts
files and needs to be compiled into JavaScript before it can run in a browser or Node.js environment. The TypeScript compiler (tsc
) is responsible for this process, translating TypeScript into plain JavaScript that is compatible with any environment that supports JavaScript.
Getting Started with TypeScript
To start using TypeScript, you'll need to install it via npm:
npm install -g typescript
// Define an interface for a Person
interface Person {
firstName: string;
lastName: string;
age: number;
greet(): string;
}
// Create a class that implements the Person interface
class Student implements Person {
firstName: string;
lastName: string;
age: number;
course: string;
constructor(firstName: string, lastName: string, age: number, course: string) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
this.course = course;
}
// Implement the greet method
greet(): string {
return `Hello, my name is ${this.firstName} ${this.lastName}, and I am ${this.age} years old.`;
}
// Additional method
getCourse(): string {
return `I am studying ${this.course}.`;
}
}
// Create an instance of the Student class
const student = new Student('John', 'Doe', 21, 'Computer Science');
// Use the methods of the Student class
console.log(student.greet()); // Output: Hello, my name is John Doe, and I am 21 years old.
console.log(student.getCourse()); // Output: I am studying Computer Science.